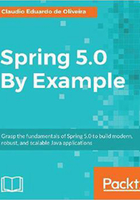
Creating the CategoryResource class
We will create our first REST resource, let's get started with the CategoryResource class which is responsible for managing our Category class. The implementation of this entity will be simple, and we will create CRUD endpoints such as create, retrieve, update, and delete. We have two important things we must keep in mind when we create the APIs. The first one is the correct HTTP verb such as POST, GET, PUT and DELETE. It is essential for the REST APIs to have the correct HTTP verb as it provides us with intrinsic knowledge about the API. It is a pattern for anything that interacts with our APIs. Another thing is the status codes, and it is the same as the first one we must follow, this is the pattern the developers will easily recognize. The Richardson Maturity Model can help us create amazing REST APIs, and this model introduces some levels to measure the REST APIs, it's a kind of thermometer.
Firstly, we will create the skeleton for our APIs. Think about what features you need in your application. In the next section, we will explain how to add a service layer in our REST APIs. For now, let's build a CategoryResource class, our implementation could look like this:
package springfive.cms.domain.resources;
import java.util.Arrays;
import java.util.List;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
import springfive.cms.domain.models.Category;
import springfive.cms.domain.vo.CategoryRequest;
@RestController
@RequestMapping("/api/category")
public class CategoryResource {
@GetMapping(value = "/{id}")
public ResponseEntity<Category> findOne(@PathVariable("id") String id){
return ResponseEntity.ok(new Category());
}
@GetMapping
public ResponseEntity<List<Category>> findAll(){
return ResponseEntity.ok(Arrays.asList(new Category(),new Category()));
}
@PostMapping
public ResponseEntity<Category> newCategory(CategoryRequest category){
return new ResponseEntity<>(new Category(), HttpStatus.CREATED);
}
@DeleteMapping("/{id}")
@ResponseStatus(HttpStatus.NO_CONTENT)
public void removeCategory(@PathVariable("id") String id){
}
@PutMapping("/{id}")
public ResponseEntity<Category> updateCategory(@PathVariable("id") String id,CategoryRequest category){
return new ResponseEntity<>(new Category(), HttpStatus.OK);
}
}
The CategoryRequest can be found at GitHub (https://github.com/PacktPublishing/Spring-5.0-By-Example/tree/master/Chapter02/src/main/java/springfive/cms/domain/vo).
We have some important concepts here. The first one is @RestController. It instructs the Spring Framework that the CategoryResource class will expose REST endpoints over the Web-MVC module. This annotation will configure some things in a framework, such as HttpMessageConverters to handle HTTP requests and responses such as XML or JSON. Of course, we need the correct libraries on the classpath, to handle JSON and XML. Also, add some headers to the request such as Accept and Content-Type. This annotation was introduced in version 4.0. It is a kind of syntactic sugar annotation because it's annotated with @Controller and @ResponseBody.
The second is the @RequestMapping annotation, and this important annotation is responsible for the HTTP request and response in our class. The usage is quite simple in this code when we use it on the class level, it will propagate for all methods, and the methods use it as a relative. The @RequestMapping annotation has different use cases. It allows us to configure the HTTP verb, params, and headers.
Finally, we have @GetMapping, @PostMapping, @DeleteMapping, and @PutMapping, these annotations are a kind of shortcut to configure the @RequestMapping with the correct HTTP verbs; an advantage is that these annotations make the code more readable.
Except for the removeCategory, all the methods return the ResponseEntity class which enables us to handle the correct HTTP status codes in the next section.