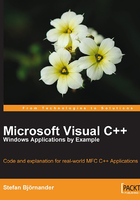
Streams and File Processing
We can open, write to, read from, and close files with the help of streams. Streams are predefined classes. ifstream
is used to read from files, and ofstream
is used to write to files. They are subclasses of istream
and ostream
in the operator overload section above. The program below reads a series of integers from the text file input.txt
and writes their squares to the file output.txt
. The stream operator returns false when there are no more values to be read from the file. Note that we do not have to close the file at the end of the program, the destructor will take care of that.
TextStream.cpp
#include <iostream> #include <fstream> using namespace std; void main(void) { ifstream inFile("Input.txt", ios::in); ofstream outFile("Output.txt", ios::out); int iValue; while (inFile >> iValue) { outFile << (iValue * iValue) << endl; } }
The text files are written in plain text and can be viewed by the editor.
Input.txt
1 2 3 4 5
Output.txt
1 4 9 16 25
We can also read and write binary data with the stream classes. The program below writes the numbers 1 to 10 to the file Numbers.bin
and then reads the same series of values from the file. The methods write
and read
take the address of the value to be read or written and the size of the value in bytes. They return the number of bytes actually read or written. When reading, we can check whether we have reached the end of the file by counting the number of read bytes; if it is zero, we have reached the end. Even though we do not have to close the file, it is appropriate to do so when the file has been written so that the values are safely saved before we open the same file for reading.
BinaryStreams.cpp
#include <iostream> #include <fstream> using namespace std; void main(void) { ofstream outFile("Numbers.bin", ios::out); for (int iIndex = 1; iIndex <= 10; ++iIndex) { outFile.write((char*) &iIndex, sizeof iIndex); } outFile.close(); ifstream inFile("Numbers.bin", ios::in); int iValue; while (inFile.read((char*) &iValue, sizeof iValue) != 0) { cout << iValue << endl; } }
The values are stored in compressed form in the binary file Numbers.bin
, which is why they are not readable in the editor. Here is a screen dump of the file:

Even though these file processing techniques are of use in many situations, we will not use them in the applications of this book. Instead, we will use the technique of Serialization, described in Chapter 3.