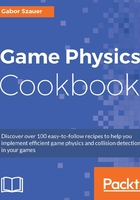
View matrix
The LookAt
function is mainly used for 3D graphics. It is a convenient way to position a 3D camera. While graphics programming is outside the scope of this book, for our math library to be practical we need to implement some graphics-related functionality.
Getting a vertex (vector) to become a pixel primarily involves three matrix transformations. The world transform, view transform, and projection transform. All three of these transformations are expressed as a matrix multiplication.
- The world transform takes the vertex from model space to world space, we've already implemented this as the
Transform
function - The view transform takes a vertex from world space and transforms it to eye space, sometimes called view space or camera space
- The projection transform takes vertices from eye space and puts them into normalized device coordinates
If we multiply a vertex by the view matrix, the vertex ends up in eye space. Eye space transforms the vertex in the world so it's relative to a camera placed at (0, 0, 0)
looking down the positive Z axis.
That is, when the camera moves, it doesn't really move through the world, the world moves around the camera. This doesn't sound very intuitive, but at least the matrix is easy enough to generate. We simply take the world space transform of the camera and invert it.
Getting ready
The Transform
function we wrote in the last section generates the World Transform matrix. In this section, we are going to write a LookAt
function, which will generate the View Transform matrix.
To find the view matrix we could create a rotation and a translation matrix, multiply them together, and invert the result. However, that would be an expensive function! Instead, we can take advantage of the fact that the inverse of an ortho-normal matrix is that same as the transpose of the matrix.
An ortho-normal matrix is a matrix whose basis vector are orthogonal and of unit length. All of the functions we have created to make rotation matrices return ortho-normal matrices.
Because of this, we can create the rotation sub-matrix transposed. That will give us the inverted rotation. To get the inverted translation, we hand code what a matrix multiplication would be, and negate the result.
How to do it…
Follow these steps to implement a function that return the view matrix of a camera given its position, the target the camera is looking, and the relative up vector:
- Add the declaration of the
LookAt
function tomatrices.h
:mat4 LookAt(const vec3& position, const vec3& target, const vec3& up);
- Implement the
LookAt
function inmatrices.cpp
:mat4 LookAt(const vec3& position, const vec3& target, const vec3& up) { vec3 forward = Normalized(target - position); vec3 right = Normalized(Cross(up, forward)); vec3 newUp = Cross(forward, right); return mat4( // Transposed rotation! right.x, newUp.x, forward.x, 0.0f, right.y, newUp.y, forward.y, 0.0f, right.z, newUp.z, forward.z, 0.0f, -Dot(right, position), -Dot(newUp, position), -Dot(forward, position), 1.0f ); }
How it works…
We need to provide the LookAt
function with enough data to build two matrices. The first matrix is the rotation of the camera, the second is the position. We can construct these matrices using three arguments:
- The position of the camera
- The position of whatever the camera is looking at
- The direction up is, usually this means world up
Based on these three vectors, we can construct a rotation basis. To obtain the forward vector we normalize the vector pointing from the cameras position to its target. To find an orthogonal vector, pointing to the right of this forward vector, we take the cross-product of the up and forward vectors. At this point we just need to construct a new up vector we can be sure is orthogonal to both forward and right, we do this by taking the cross product of the forward and right vectors.
These three vectors make up the rotation basis for the camera. The right vector is the first row of the rotation matrix, the up vector is the second, and the right vector is the last row. If we multiply this rotation matrix with a translation matrix acquired from the position parameter, we can find the world matrix of the camera.
Instead of the world matrix of the camera, we want its view matrix. The view matrix is the inverse of the camera's world matrix. To obtain the view matrix, we transpose the rotation part of the matrix. We then negate the dot product of each axis with the position of the camera. The dot product operation produces the same result as multiplying a translation and a rotation matrix together. We negate this value to get the inverse translation.