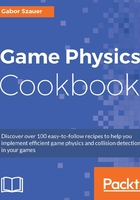
Operations on a 4x4 matrix
We know how to find the minor, cofactor, and determinant of 2 X 2 and 3 X 3 matrices. In this section, we're going to implement those functions for a 4 X 4 matrix. We begin with the matrix of minors. The process for finding the minor of element i, j in a 4 X 4 matrix is the same as it was for a 3 X 3 matrix. We eliminate row i and column j of the matrix, the determinant of the resulting 3 X 3 matrix is the minor for element i, j.
Next, we find the cofactor. To find the cofactor we just follow the same formula we did for the 3 X 3 matrix:

To get the cofactor of element i, j, we take the minor of that element and multiply it by . Finally, we have to find the determinant of the matrix. Again, we do this by following the same formula we used for the 3 X 3 matrix:

To find the determinant, we loop through any row of the matrix and sum up the result of multiplying each of the elements in the row by their respective cofactor. You only need to loop through one row, and which row it is does not matter. By convention i will loop through the first row in this book.
Getting ready
In order to find the minor of a 4 X 4 matrix, we have to implement a Cut
function. This function will cut a 3 X 3 matrix from a 4 X 4 matrix by eliminating a row and a column. This will work similarly to the Cut
function we already implemented that cuts a 2 X 2 matrix from a 3 X 3 matrix. Once the Cut
function is created, the rest of the functions will be easy to implement; they will be very similar to their 3 X 3 matrix counterparts.
How to do it…
Follow these steps to write the 4 X 4 versions of the Cut
, Minor
, Cofactor
and Determinant
functions which we already implemented for 3 X 3 matrices:
- Add the declaration for all the 4 X 4 matrix functions we need to implement to
matrices.h
:mat3 Cut(const mat4& mat, int row, int col); mat4 Minor(const mat4& mat); mat4 Cofactor(const mat4& mat); float Determinant(const mat4& mat);
- Let's first implement the
Cut
function. This function is going to cut a 3 X 3 matrix from a 4 X 4 matrix by eliminating one row and one column:mat3 Cut(const mat4& mat, int row, int col) { mat3 result; int index = 0; for (int i = 0; i < 4; ++i) { for (int j = 0; j < 4; ++j) { if (i == row || j == col) { continue; } int target = index++; int source = 4 * i + j; result.asArray[target] = mat.asArray[source]; } } return result; }
- Using the newly created
Cut
function, implement the 4 X 4 version of theMinor
function inmatrices.cpp
:mat4 Minor(const mat4& mat) { mat4 result; for (int i = 0; i <4; ++i) { for (int j = 0; j <4; ++j) { result[i][j] = Determinant(Cut(mat, i, j)); } } return result; }
- With the newly created
Minor
function, we can create the 4 X 4 version of theCofactor
function. Like its 3 X 3 counterpart, this function is going to call the genericCofactor
function with appropriate arguments:mat4 Cofactor(const mat4& mat) { mat4 result; Cofactor(result.asArray, Minor(mat).asArray, 4, 4); return result; }
- Finally, implement the 4 X 4 determinant function in
matrices.cpp
:float Determinant(const mat4& mat) { float result = 0.0f; mat4 cofactor = Cofactor(mat); for (int j = 0; j < 4; ++j) { result += mat.asArray[4 * 0 + j] * cofactor[0][j]; } return result; }
How it works…
The minor, cofactor, and determinant functions of a 4 X 4 matrix follow the same formula as those of a 3 X 3 and 2 X 2 matrix. If the formulas are the same, why did we wait until now to implement the 4 X 4 versions of these functions, instead of implementing them earlier with the lower order versions? Because these functions are mathematically recursive.
In order to find the determinant of a 4 X 4 matrix, you need to know its cofactor. In order to find the cofactor of a 4 X 4 matrix, you need to know its minor. In order to find the minor of a 4 X 4 matrix, you need to be able to solve the determinant of a 3 X 3 matrix. This pattern continues until you need to be able to find the determinant of a 2 X 2 matrix! The formulas we've covered so far will work for any higher order matrix, so long as you know how to solve them for all lower order matrices.