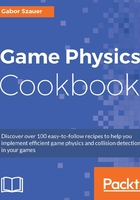
Determinant of a 2x2 matrix
Determinants are useful for solving systems of linear equations; however, in the context of a 3D physics engine, we use them almost exclusively to find the inverse of a matrix. The determinant of a matrix M is a scalar value, it's denoted as .The determinant of a matrix is the same as the determinant of its transpose
.
We can use a shortcut to find the determinant of a 2 X 2 matrix; subtract the product of the diagonals. This is actually the manually expanded form of Laplace Expansion; we will cover the proper formula in detail later:

One interesting property of determinants is that the determinant of the inverse of a matrix is the same as the inverse determinant of that matrix:

Finding the determinant of a 2 X 2 matrix is fairly straightforward, as we have already expanded the formula. We're just going to implement this in code.
How to do it…
Follow these steps to implement a function which returns the determinant of a 2 X 2 matrix:
- Add the declaration for the determinant function to
matrices.h
:float Determinant(const mat2& matrix);
- Add the implementation for the determinant function to
matrices.cpp
:float Determinant(const mat2& matrix) { return matrix._11 * matrix._22 – matrix._12 * matrix._21; }